CODE CONDITION IMPLEMENTATION: |
1. Make the following changes for IfCritical, i.e. give the condition property to ‘code condition’. |
|
Figure 8
|
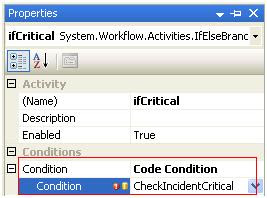 |
2. This will give an additional property called condition. If you open the view-code for workflow1.cs, the name ‘CheckIncidentCritical’, will be the event names that visual studio automatically generates for you. |
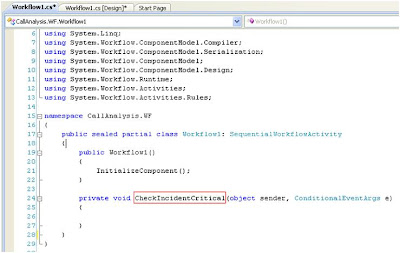 |
Figure 9
|
3. Make the changes for IfHigh and IfMedium, similar to IfCritical and name the events CheckIncidentHigh and CheckIncidentMedium respectively. |
4. For IfIncident too select condition as ‘code condition’ and name the condition property as ‘CheckIncident’. Now do following changes to code |
public sealed partial class Workflow1: SequentialWorkflowActivity { public Workflow1(){ InitializeComponent(); } private void CheckIncidentCritical(object sender, ConditionalEventArgs e){ Console.WriteLine("Incident-Critical Reached"); } private void CheckIncidentHigh(object sender, ConditionalEventArgs e){ Console.WriteLine("Incident-High Reached"); e.Result = true; } private void CheckIncidentMedium(object sender, ConditionalEventArgs e) { Console.WriteLine("Incident-Medium Reached"); } private void CheckIncident(object sender, ConditionalEventArgs e){ Console.WriteLine("Incident Main Reached"); e.Result = true; } }
|
5. Let’s have our first compilation. Right click the project and select build. Solution should compile without any error.
|
6. Now we are ready for our first run. Open the file ‘Program.cs’, it is the file that is currently helping us to be the Host application. Put a break-point on line ‘instance.start()’ as shown below.
|
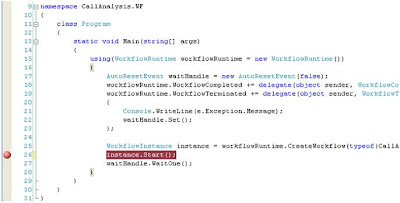 |
Figure 10
|
7. Right click the solution and select “debug->start a new instance”. Press F10 until reach next line. Check the console window. Output looks like this
|
OUTPUT: |
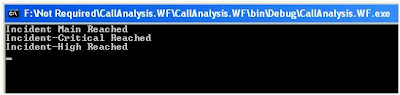 |
Figure 11
|
Explanation: |
1. Ifincident will evaluate to true because of e.result=true in following line of code
|
private void CheckIncident(object sender, ConditionalEventArgs e){ Console.WriteLine("Incident Main Reached"); e.Result = true; }
|
2. Control goes to ‘ifcritical’ where e.result is not set and bool’s default value ‘false’ is taken. Print statement executed.
|
3. Control goes to ‘Ifhigh’, here e.result is set to true. Print statement executed. |
4. Since no more statements follow it. Execution is stopped.
|
I know here you must be thinking, there seems no big difference since false and true both resulted in printing. So let’s get into little more coding with reading values from input window and seeing the difference. For that do the following changes to design. |
1. Add a code activity (refer figure4 to add a control from toolbox to workflow) for each of the ifcritical, ifhigh and ifMedium activity and associate the code with it such that following changes happen in Design(figure12) and Code(figure13) respectively.
|
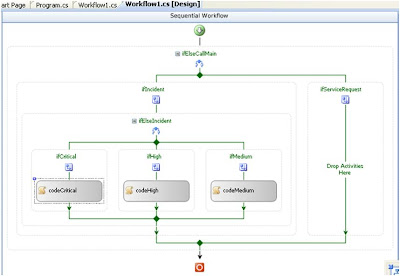 |
Figure 12
|
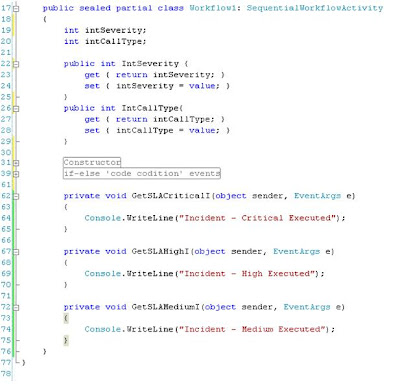 |
Figure 13 |
|
Subhanallah. Sairam. Good blog. i am indonesian ( Java etnic ), i have a friend from india, his name is Anil Ravuri.
ReplyDelete